Destructuring TypeScript Objects
- AndyH
- Mar 17, 2019
- 1 min read
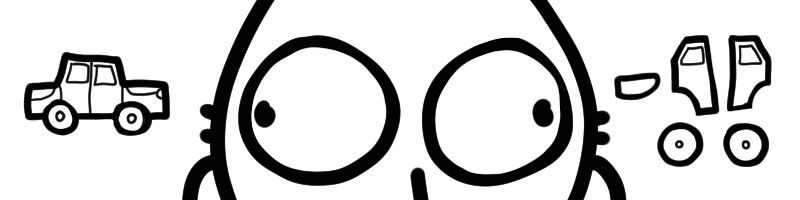
I've seen some example code in TypeScript that initially got me scratching my head. After a quick search on Google, it turns out that TypeScript has a neat trick called destructuring which extracts properties you need from an object and assigns them to variables.
Take this class for example:
class Car { constructor( public make: string, public colour: string, public doors: number) { } }
I can create a car object:
let bobCar = new Car( "Honda", "Red", 5 ); let fredCar = new Car( "Ford", "Silver", 3 );
And I can assign variables a property from these objects:
let carMake: string = bobCar.make; let carDoors: number = bobCar.doors; let carColour: string = fredCar.colour;
With destructuring I can create variables while assigning them with the properties from the object:
let { make: carMake, door: carDoors } = bobCar; let { colour: carColour } = fredCar;
I now have three variables called carMake, carDoors and carColour loaded with the properties from my objects. If you want to create variables with the same name as the properties it gets even easier:
let { make, door } = bobCar; let { colour } = fredCar;
I now have three variables called make, door and colour. Easy!
Komentarze