Working with State in a React Component
- AndyH
- Mar 19, 2019
- 2 min read
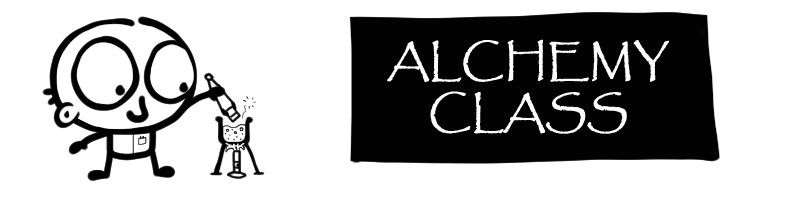
Very early on you will want to use the state to store and use dynamic data in your React component.
In this example I want to take a different course of action in the web part if the user is an approver. I could use a property instead and work out if the user is an approver in the parent component but for the sake of a demonstration I'm using State. It could be that I create a link or button to a document or to start off a process but for simplicity in this example I'm going to display one of two messages:
Yay - approver!
You're not the approver I'm looking for!
To facilitate this, I have an approver variable defined in the IState interface and this is where we will store a value of true if the current user is an appover, or false if not:
export interface IState { approver: boolean; }
The IState interface is passed as the second parameter of the React.Component in the components class so it knows what our state should look like:
export default class MyComponent extends React.Component< {}, IState >
The approver state variable is currently undefined. A constructor is required to initialise the state which I do by assigning a default value of false, but we're told best not to load data from SharePoint or other data source in here:
public constructor( props: IApproveServiceTowerProps ) { super( props ); this.state = { approver: false }; }
Instead, to safely load the data, componentDidMount should be used:
public componentDidMount(){ this._getApprover(); }
I'm just going to set the approver state in this example, but this is where you can use PnPJS to retrieve information from SharePoint to make this decision:
private _getApprover() : void { this.setState( { approver: true } ); }
Note that setState is used to change the approver state value outside of the constructor.
Finally in the render method, we can refer to and use the approver state:
public render(): React.ReactElement< {} > { const { approver } = this.state; if ( approver && approver === true ) { return <div>Yay - approver!</div>; } else { return <div>You're not the approver we're looking for!</div>; } }
I use destructuring to create a local approver variable in the render method which is perhaps overkill here, but good to keep in mind when there is more going on in your state.
Comments